Analog I/O
Part 1
The first part of this lab was to create a simple analog input with varying output. For mine, I used a pressure sensor, a potentiometer, and NeoPixels to create a rudimentary strong man device. The number of lights corresponded to the pressure and the color was changed by rotating the potentiometer.
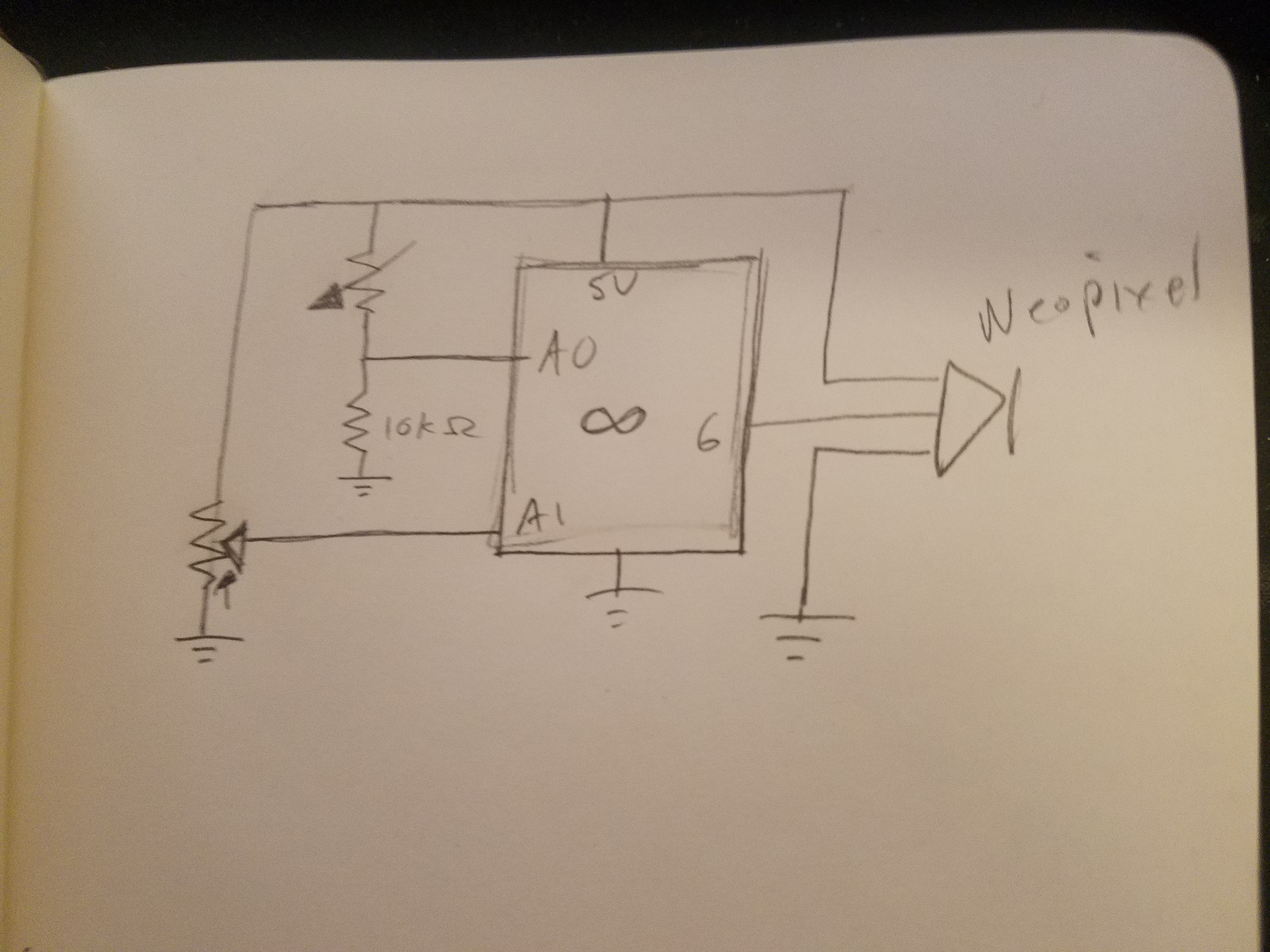
I adopted the WheelPosition function from the AdaFruit
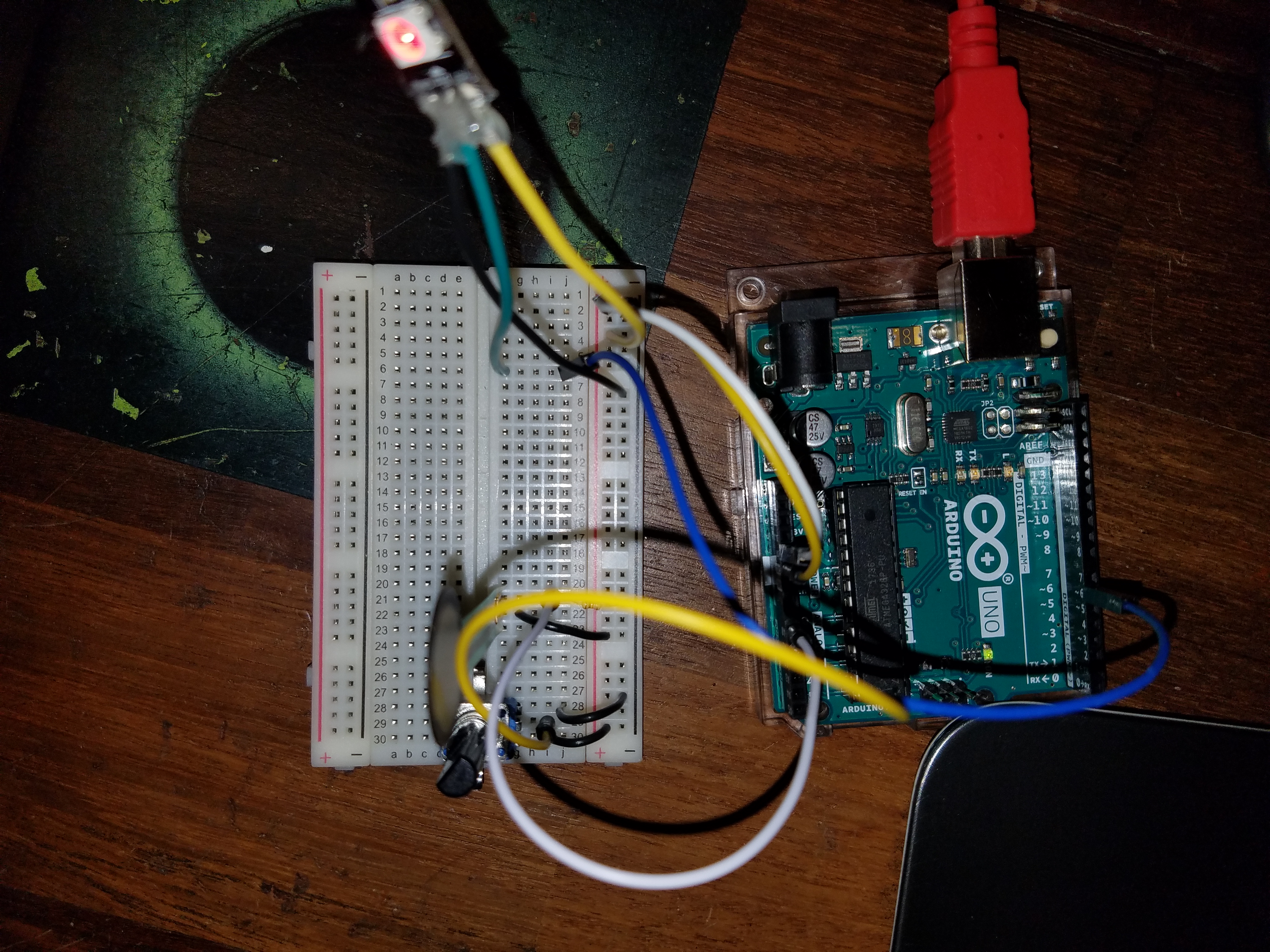
Part 2
In the second part, we used an analog input to control the tone of a speaker. I found the random buzzing that was created to be annoying so I changed it to notes.
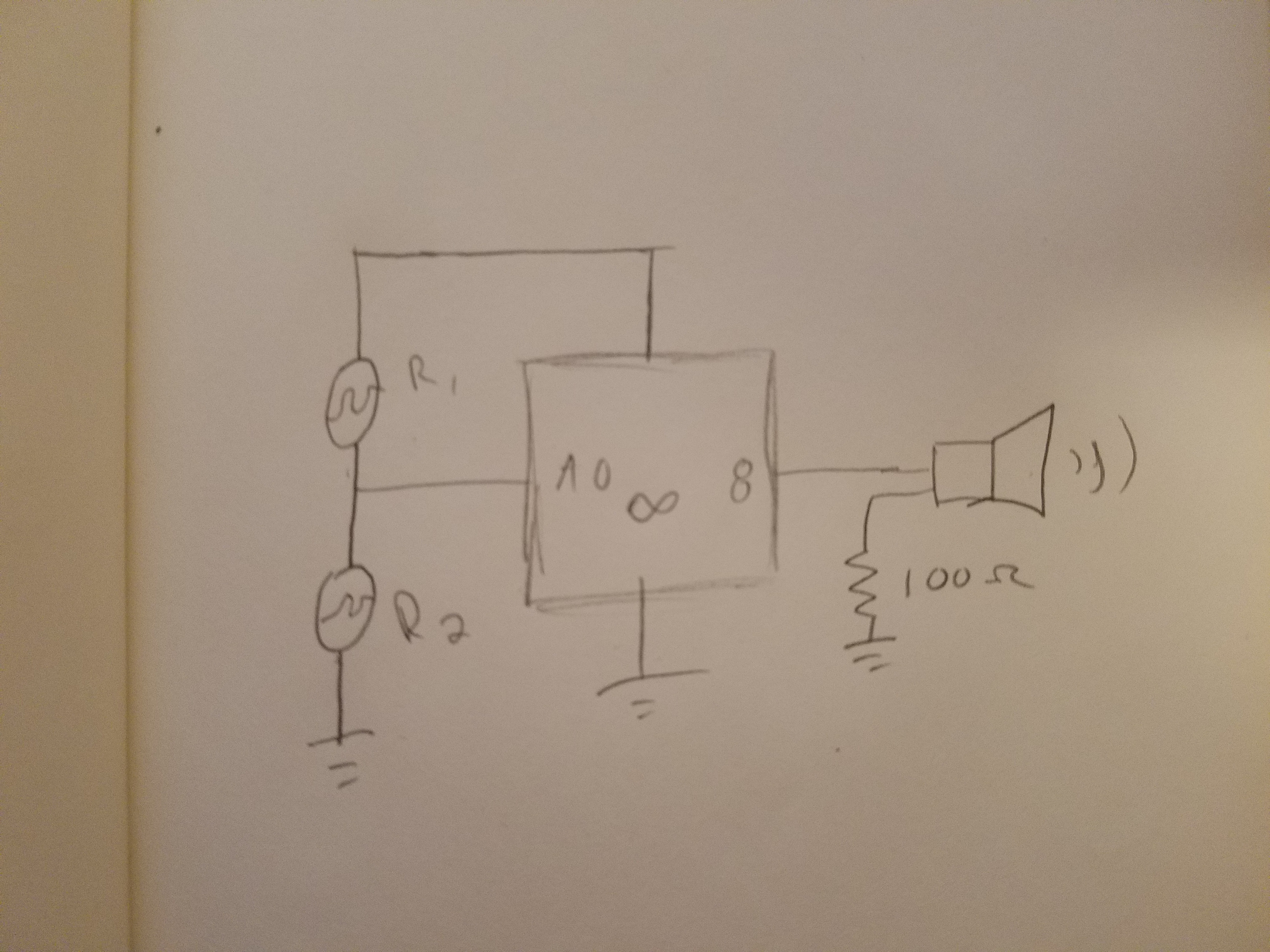
The note values were taken from Wikipedia.
For this project, I made a box from plywood. Unfortunately, I was unable to locate my jigsaw and had to use a circular saw (which was too large for such a small project) and a
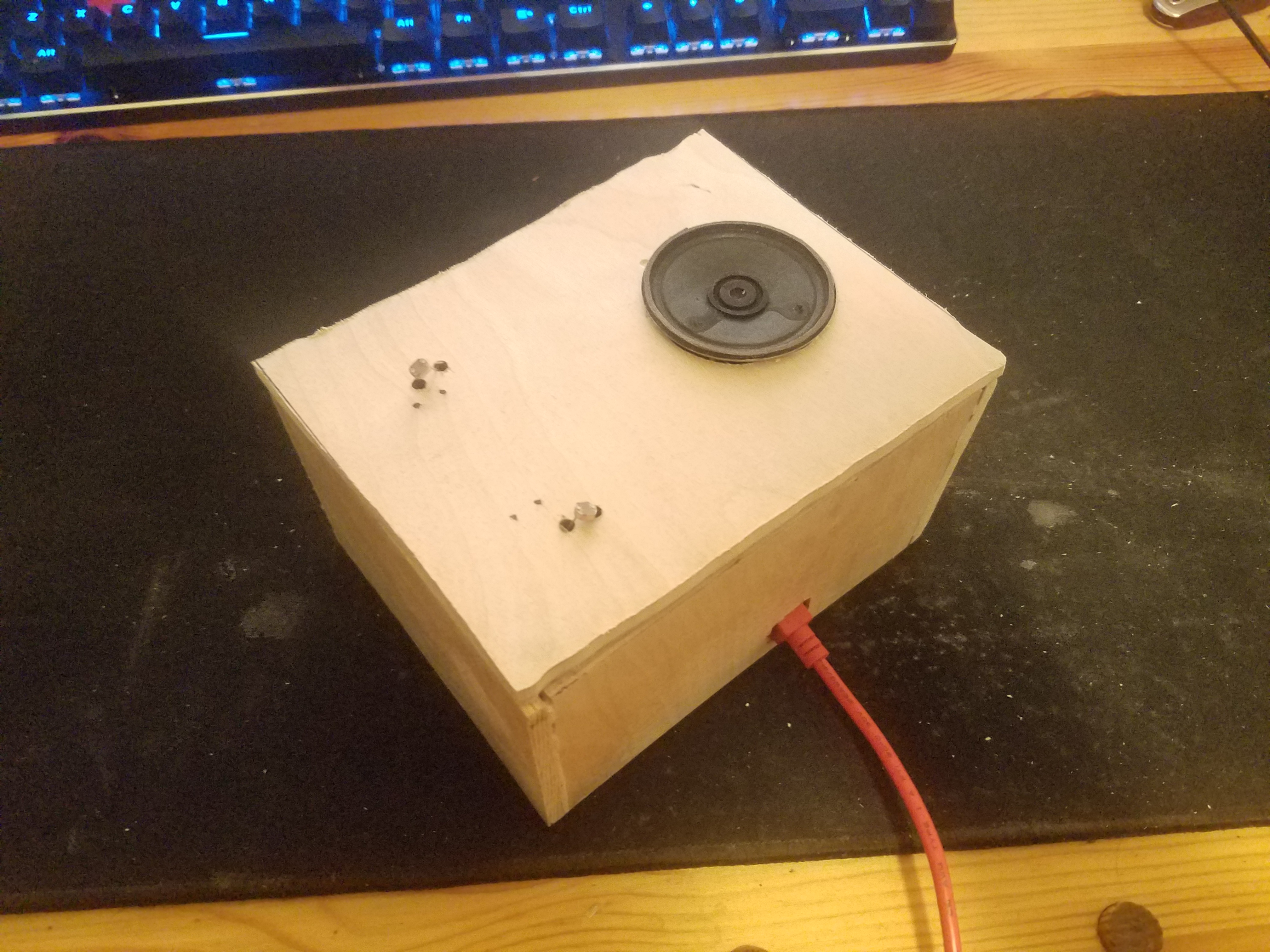